Table of Contents
- Getting Started
- EO.Pdf
- Overview
- Installation and Deployment
- Using HTML to PDF
- Using PDF Creator
- Using PDF Creator
- Getting Started
- Advanced Formatting Techniques
- Interactive Features
- Low Level Content Objects
- Working with Existing PDF Files
- Using in Web Application
- Advanced Topics
- EO.Web
- EO.WebBrowser
- EO.Wpf
- Common Topics
- Reference
Floating Blocks |
Overview
A block content can be "floated". When it is floated, it is "pinned" to a specific spot on the page and allows other inline contents (typically text) to flow around it. The following image demonstrates the typical result of a floating block:
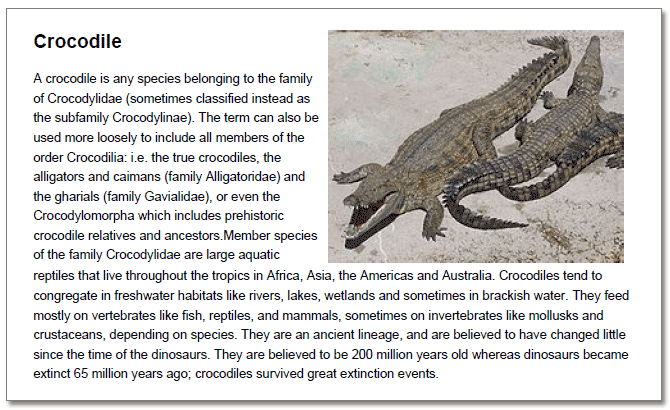
Float a Block Content
Note: All floating blocks must appear before any non-floating contents in the content tree. If a block is set to float but a non-floating content has appeared before this block, then floating settings on this block will be ignored.
To float a block, set the block's Top or Left style property, or both. For example, the following code floats the block towards the right and reserves 2 inches on the left side.
block.Style.Left = 2f;
Note: The parent content of the floated block content must also be a block content. Otherwise an error will be thrown.
The following diagram illustrates the result of the above code:
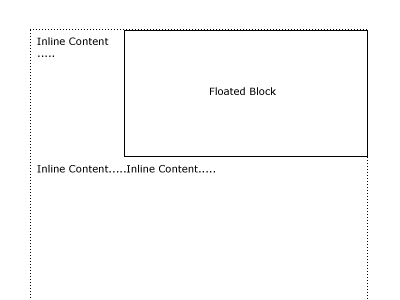
Because the code did not set the block's Width, the block takes the maximum available width and reaches the right edge of the available space on the page.
The following code floats the block to the left:
block.Style.Left = 0f; block.Style.Width = 2f;
The above code floats the block towards left and leaves all the available space on the right side available for subsequent inline contents. If the block's Left was not set, then while the block's Width would still be 2 inches, extra space on the right side of the block would have not been used by subsequent inline contents because the block wouldn't be floated.
The following diagram illustrates the result of the above code:
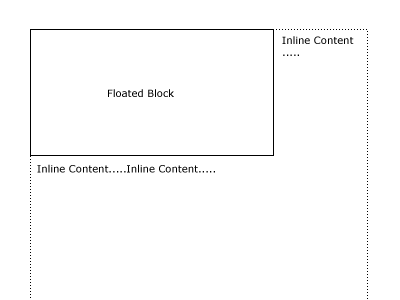
The following code floats the block in the middle:
block.Style.Left = 2f; block.Style.Width = 2f;
Because it has Left set, it reserves space on the left side. Also because it has Width set, extra space on the right side (assume the page is more than 4 inches wide) will also be used by inline contents.
The following diagram illustrates the result of the above code:
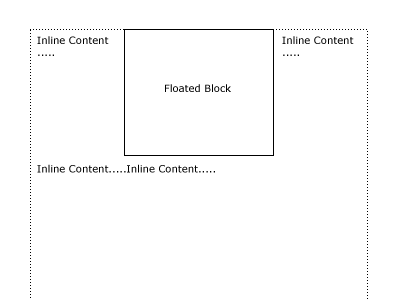
The Top style property can be used in the same way and together with Left property to float a block in the middle of a page:
block.Style.Top = 2f; block.Style.Left = 2f; block.Style.Width = 2f; block.Style.Height = 2f;
The following diagram illustrates the result of the above code:
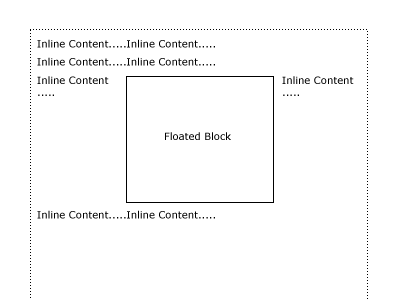
You can not float a block to the bottom of the page.
Float an Inline Content
You can not float an inline content directly. However you can always place the inline content inside a block content, then float that block instead. The following code floats an image in the document:
//Load the image System.Drawing.Image gdiImage = System.Drawing.Image.FromFile("c:\\hello.gif"); //Create an AcmImage object AcmImage image = new AcmImage(gdiImage); //Create an AcmBlock object to hold the AcmImage object AcmBlock block = new AcmBlock(); block.Children.Add(image); //Float the block block.Style.Left = 2f; block.Style.Width = 2f;
Scope of Floated Contents
A block's floating scope is always its parent block, or the page in case a parent block does not exist. Both Left and Top property are relative to the left edge or the top edge of its parent block's (or the page) client area.
It is possible to float multiple blocks inside the same block. For example, one floats to the left, another one floats to the right. However all floating blocks must appear before any non-floating contents in the content tree. If a block is set to float but a non-floating content has appeared before this block, then floating settings on this block will be ignored.